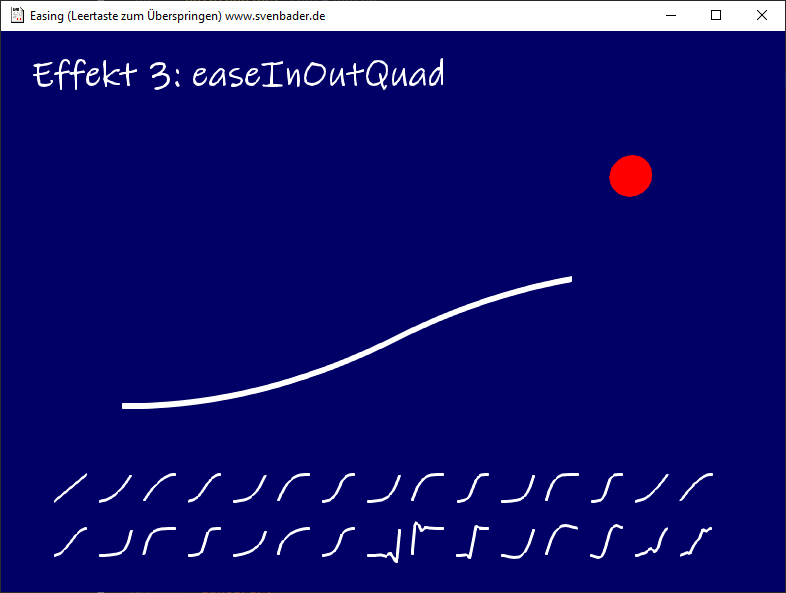
Herunterladen
Da ich solche Effekte häufiger verwende, habe ich sie nun in einer Include zusammengefasst und nach dem jQuery-Vorbild noch einige hinzugefügt.
Die Idee ist, dass die Bewegung eines Sprites (oder auch Menüelements etc.) nicht linear verläuft, sondern am Anfang und/oder Ende geglättet wird.
Der Beispielcode ist wegen der Visualisierung etwas länger geworden, die Verwendung selbst ist aber eigentlich ein Zweizeiler:
Man muss lediglich dafür sorgen, dass der Parameter (hier step!) in konstanten Schritten von 0 bis 1 zählt. Die Rückgabe sind auch wieder Werte von 0 (Animationsanfang) bis 1 (Animationsende) - nur eben dazwischen nicht linear.
Die Include Datei (ease.inc)
'Basiert auf jQuery unter der MIT Lizenz (https://jquery.org/license/)
'
'XProfan-Version von Sven Bader 2021 www.svenbader.de
Declare Pi!, c1!, c2!, c3!, c4!, c5!, c6!
PI! = Pi()
c1! = 1.70158
c2! = c1! * 1.525
c3! = c1! + 1.0
c4! = (2 * PI!) / 3.0
c5! = (2 * PI!) / 4.5
c6! = PI! * 0.5
Proc bounceOut
parameters x!
declare n1!,d1!
n1! = 7.5625
d1! = 2.75
if (x! < 1.0/d1!)
return n1! * x! * x!
elseif (x! < (2.0/d1!))
x! = x!-(1.5/d1!)
return (n1! * x! * x! + 0.75)
elseif (x! < 2.5/d1!)
x! = x!-(2.25/d1!)
return (n1! * x! * x! + 0.9375)
else
x! = x!-(2.625/d1!)
return (n1! * x! * x! + 0.984375)
endif
Endproc
Proc Linear
parameters x!
return x!
EndProc
Proc easeInQuad
parameters x!
return (x! * x!)
EndProc
Proc easeOutQuad
parameters x!
return (1.0 - (1.0 - x!) * (1.0 - x!))
EndProc
Proc easeInOutQuad
parameters x!
return if(x! < 0.5, (2.0 * x! * x!), (1.0 - (-2.0 * x! + 2.0)^2 * 0.5))
EndProc
Proc easeInCubic
parameters x!
return (x! * x! * x!)
EndProc
Proc easeOutCubic
parameters x!
return (1.0 - (1.0 - x!)^3)
EndProc
Proc easeInOutCubic
parameters x!
return if(x! < 0.5, (4 * x! * x! * x!), (1.0 - (-2.0 * x! + 2.0)^3 * 0.5))
EndProc
Proc easeInQuart
parameters x!
return (x! * x! * x! * x!)
EndProc
Proc easeOutQuart
parameters x!
return (1.0 - (1.0 - x!)^4)
EndProc
Proc easeInOutQuart
parameters x!
return if(x! < 0.5, (8 * x! * x! * x! * x!), (1.0 - (-2.0 * x! + 2.0)^4 * 0.5))
EndProc
Proc easeInQuint
parameters x!
return (x! * x! * x! * x! * x!)
EndProc
Proc easeOutQuint
parameters x!
return (1 - (1 - x!)^5)
EndProc
Proc easeInOutQuint
parameters x!
return if(x! < 0.5, (16.0 * x! * x! * x! * x! * x!), (1.0 - (-2.0 * x! + 2)^5 * 0.5))
EndProc
Proc easeInSine
parameters x!
return 1.0 - cos(x! * c6!)
EndProc
Proc easeOutSine
parameters x!
return sin(x! * c6!)
EndProc
Proc easeInOutSine
parameters x!
return (-(cos(PI! * x!) - 1.0) * 0.5)
EndProc
Proc easeInExpo
parameters x!
return if((x! = 0.0), 0.0, 2^(10.0 * x! - 10.0))
EndProc
Proc easeOutExpo
parameters x!
return if(x! = 1.0, 1.0, (1.0 - 2^(-10.0 * x!)))
EndProc
Proc easeInOutExpo
parameters x!
return if(x! = 0.0, 0.0, if(x! = 1.0, 1.0, if(x! < 0.5, (2^(20 * x! - 10) * 0.5), (2 - 2^(-20.0 * x! + 10.0)) *0.5)))
EndProc
Proc easeInCirc
parameters x!
return 1.0 - sqrt(1.0 - x!^2)
EndProc
Proc easeOutCirc
parameters x!
return sqrt(1.0 - (x! - 1.0)^2)
EndProc
Proc easeInOutCirc
parameters x!
return if(x! < 0.5, ((1.0 - sqrt(1.0 - (2.0 * x!)^2)) * 0.5), \
((sqrt(1.0 - (-2.0 * x! + 2.0)^2) + 1.0) * 0.5))
EndProc
Proc easeInElastic
parameters x!
return if(x! = 0.0, 0.0, if(x! = 1.0, 1.0, -(2^(10.0 * x! - 10.0) * sin((x! * 10.0 - 10.75) * c4!))))
EndProc
Proc easeOutElastic
parameters x!
return if(x! = 0, 0, if(x! = 1.0, 1.0, (2^(-10.0 * x!) * sin((x! * 10 - 0.75) * c4!) + 1.0)))
EndProc
Proc easeInOutElastic
parameters x!
return if(x! = 0, 0, if(x! = 1.0, 1.0, if(x! < 0.5, (-(2^(20 * x! - 10) * sin((20 * x! - 11.125) * c5!)) * 0.5), \
(2^(-20.0 * x! + 10.0) * sin((20.0 * x! - 11.125) * c5!) * 0.5 + 1.0))))
EndProc
Proc easeInBack
parameters x!
return c3! * x! * x! * x! - c1! * x! * x!
EndProc
Proc easeOutBack
parameters x!
return 1 + c3! * (x! - 1)^3 + c1! * (x! - 1)^2
EndProc
Proc easeInOutBack
parameters x!
return if(x! < 0.5, (((2 * x!)^2 * ((c2! + 1.0) * 2.0 * x! - c2!)) * 0.5), \
(((2.0 * x! - 2.0)^2 *((c2! + 1) * (x! * 2.0 - 2.0) + c2!) + 2) * 0.5))
EndProc
Proc easeInBounce
parameters x!
return 1.0 - bounceOut(1.0 - x!)
EndProc
Proc easeInOutBounce
parameters x!
return if(x! < 0.5, ((1.0 - bounceOut(1.0 - 2.0 * x!)) * 0.5), ((1.0 + bounceOut(2.0 * x! - 1.0)) * 0.5))
EndProc
Ein Beispiel-Listing (ease.prf)
'XProfan-Version von Sven Bader 2021 www.svenbader.de
$I ease.inc
declare xpos!, ypos!, zpos!
declare font&, fontHandle&, names$, step!, effect&, step&, steps![29,200],loop&,cache&
proc DrawGLScene
oGL("Clear")
'Ball
oGL("Origin", -2+xpos!*4, 1.0, -5)
oGL("Color", 1.0,0.0,0.0,1)
oGL("Sphere", 0.15,20,20)
'Großer Graph
oGL("Origin", -2, -0.7, -5)
oGL("Color", 1.0,1.0,1.0,1)
oGL("glLineWidth", 6.0)
whileloop 0, step&-2,2
oGL("glBegin", ~GL_LINES)
oGL("glVertex2f",&loop*0.02,steps![effect&,&loop])
oGL("glVertex2f",(&loop+2)*0.02,steps![effect&,&loop+2])
oGL("glEnd")
endwhile
'Kleine Graphen (+Cachen)
ifnot cache&
cache& = ogl("Startlist")
oGL("glLineWidth", 3.0)
whileloop 0, 29
loop& = &loop
oGL("Origin", -2.5+(if(loop&>14,&loop-15,&loop)*0.33), -1.4+if(loop&>14,-0.4,0), -5)
whileloop 0, 180,20
oGL("glBegin", ~GL_LINES)
oGL("glVertex2f",&loop*0.0012,steps![loop&,&loop]*0.2)
oGL("glVertex2f",(&loop+20)*0.0012,steps![loop&,&loop+20]*0.2)
oGL("glEnd")
endwhile
endwhile
ogl("Endlist")
endif
ogl("DrawList", cache&)
'Text:
oGL("Origin", -5*(%maxx/%maxy),5 ,-15)
oGL("color", 1.0,1.0,1.0,1.0)
oGL("Print",font&,"Effekt "+str$(effect&)+": "+substr$(names$,effect&+1,","))
oGL("Show")
Endproc
windowstyle 1+2+4+8+16
windowtitle "Easing (Leertaste zum Überspringen) www.svenbader.de"
window 100,100 - 800,600;0
oGL("Init", %hWnd, 0, 0, 0.4, 0)
oGL("Posmode",1)
fontHandle&=create("Font","Ink Free",12,0,1,0,0)
font&=oGL("OutlineFont",fontHandle&,0)
Deleteobject fontHandle&
names$ = "Linear,easeInQuad,easeOutQuad,easeInOutQuad,easeInCubic,easeOutCubic,easeInOutCubic (Swing),easeInQuart,easeOutQuart,easeInOutQuart,easeInQuint,easeOutQuint,easeInOutQuint,easeInSine,easeOutSine,easeInOutSine,easeInExpo,easeOutExpo,easeInOutExpo,easeInCirc,easeOutCirc,easeInOutCirc,easeInElastic,easeOutElastic,easeInOutElastic,easeInBack,easeOutBack,easeInOutBack,easeInBounce,easeInOutBounce"
WhileNot iskey(27)
sleep 2
SELECT effect&
CASEOF 0 : xpos! = Linear(step!)
CASEOF 1 : xpos! = easeInQuad(step!)
CASEOF 2 : xpos! = easeOutQuad(step!)
CASEOF 3 : xpos! = easeInOutQuad(step!)
CASEOF 4 : xpos! = easeInCubic(step!)
CASEOF 5 : xpos! = easeOutCubic(step!)
CASEOF 6 : xpos! = easeInOutCubic(step!)
CASEOF 7 : xpos! = easeInQuart(step!)
CASEOF 8 : xpos! = easeOutQuart(step!)
CASEOF 9 : xpos! = easeInOutQuart(step!)
CASEOF 10 : xpos! = easeInQuint(step!)
CASEOF 11 : xpos! = easeOutQuint(step!)
CASEOF 12 : xpos! = easeInOutQuint(step!)
CASEOF 13 : xpos! = easeInSine(step!)
CASEOF 14 : xpos! = easeOutSine(step!)
CASEOF 15 : xpos! = easeInOutSine(step!)
CASEOF 16 : xpos! = easeInExpo(step!)
CASEOF 17 : xpos! = easeOutExpo(step!)
CASEOF 18 : xpos! = easeInOutExpo(step!)
CASEOF 19 : xpos! = easeInCirc(step!)
CASEOF 20 : xpos! = easeOutCirc(step!)
CASEOF 21 : xpos! = easeInOutCirc(step!)
CASEOF 22 : xpos! = easeInElastic(step!)
CASEOF 23 : xpos! = easeOutElastic(step!)
CASEOF 24 : xpos! = easeInOutElastic(step!)
CASEOF 25 : xpos! = easeInBack(step!)
CASEOF 26 : xpos! = easeOutBack(step!)
CASEOF 27 : xpos! = easeInOutBack(step!)
CASEOF 28 : xpos! = easeInBounce(step!)
CASEOF 29 : xpos! = easeInOutBounce(step!)
ENDSELECT
inc step&
step! = step! + 0.005
steps![effect&,step&] = xpos!
if (step! >= 1.0) OR Iskey(32)
sleep if(Iskey(32), 200, 500)
inc effect&
case cache& : ogl("DeleteList",cache&)
clear step!, step&, xpos!, cache&
case effect& = 30 : effect& = 0
endif
DrawGLScene()
EndWhile
|